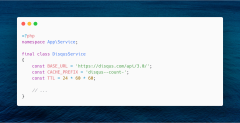
I've seen some begginer programmers asking themselves: why do I even need constants?
Variables I get, they're super important, but why have an extra thing that's like a variable, but worse?
It can't even change! And if I know that const NUMBER_OF_COLUMNS = 3
, why can't I just write 3
?
Well, first of all, it's not always just a simple 3
. Sometimes, for example, a constant is 3.141592653589793...
.
In this case writing Math::PI
is not only shorter, prettier, and more meaningful,
but usually more accurate (how many digits of π can you write down? your standard library knows a lot of them)
and not prone to typos (misspelled constant name just won't compile...).
let circ1 = 2 * Math.PI * radius;
let circ2 = 2 * 3.141582653589793 * radius;
The first line better resembles the well-known mathematical formula, and it doesn't require googling (or remembering) the actual value. The second one? Apart from being ugly, it's also wrong. Look closely.
Creating a constant with some value gives this value a label. Gives it an extra meaning.
It's not just a three anymore. Now it's the NUMBER_OF_COLUMNS
(that just happens to be three).
Now it's a three that's different from other threes.
Let's say you have 3
columns of data updated every 3
minutes. Your code is full of threes.
After coming back to your code after a break, you don't know anymore what those threes mean, they're just some numbers.
If one day you have to change the layout to two columns, how do you know which threes to replace with twos,
and which ones to keep?
Do you have to look through your entire codebase, read it and understand it?
Or maybe instead you have NUMBER_OF_COLUMNS
columns of date updated every UPDATE_INTERVAL
minutes?
const NUMBER_OF_COLUMNS = 3;
const UPDATE_INTERVAL = 3;
Easy to understand wherever they are used, and super easy to change in just a single place.
Constants are only constant during the execution. During development – the actually make changes easier.
And yes, you can do “less” with them, but that property actually adds value. It lets the programmers (and the compiler) know that it's not supposed to change. It prevents your app from being inconsistent because someone accidentally overwrote a variable.
So there you go: constants, although less flexible, are just as useful as variables.